Menu
MENU
C - Even or Odd Number
Problem Statement
Write a program in C to check whether an integer number entered by the user is Even or Odd.
Brief Description
According to the problem statement we have to write a program in C that will take an integer value as input from the user through the keyboard and will check whether it is even or odd.
An integer number which is exactly divisible by 2 is an even number otherwise it is odd.
In other words when an integer number is divided by 2 and as remainder 0 is obtained then that is an even number otherwise that is an odd number.
For example, if the user enters 8 as input, the program will generate an output indicating that 8 is an even number. But if the user enters 5 as input, the program will generate an output indicating that 5 is an odd number.
To view Flowchart click the link given below:
Program
#include
void main()
{
int num;
printf("Enter a number: ");
scanf("%d",&num);
if(num%2==0)
printf("%d is an Even number",num);
else
printf("%d is an Odd number",num);
}
Output
When the program given above is executed using Dev-C++ IDE we get the following outputs:
Output 1
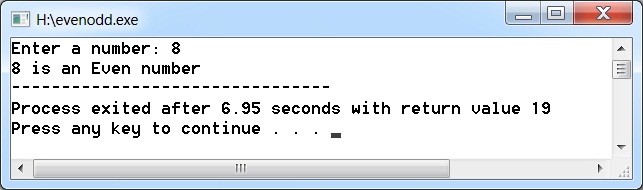
Output 2
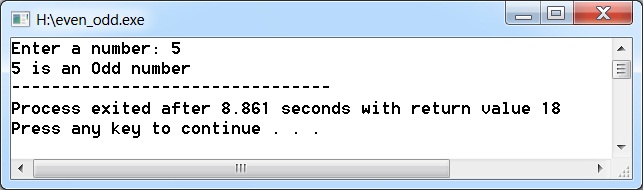
Explanation
In this program:
- An integer variable namely num is declared.
- The integer value entered by the user is stored in num.
- If an even number is entered by the user, the condition num%2==0 is evaluated to 1(True) and the statement written within if block is executed to display a message indicating that the entered number is an even number.
- If an odd number is entered by the user, the condition num%2==0 is evaluated to 0(False) and control goes to the else block and the statement written within else block is executed to display a message indicating that the entered number is an odd number.Â
When num contains 8, the condition num%2==0 is evaluated to 1(True) and the message “8 is an Even number” is displayed. The condition num%2==0 is evaluated as follows:
num | num%2==0 |
---|---|
8 | 8%2==0 ↓ 0==0 ↓ 1(True) |
When num contains 5, the condition num%2==0 is evaluated to 0(False) and the message “5 is an Odd number” is displayed. The condition num%2==0 is evaluated as follows:
num | num%2==0 |
---|---|
5 | 5%2==0 ↓ 1==0 ↓ 0(False) |
Share this page on