C - Add Two Numbers
Problem Statement
Brief Description
According to the problem statement we have to write a program in C that will take two numbers as input from the user through the keyboard and will add the two given numbers and will find the sum.
The two numbers given by the user may be integers like 5, 8, 12 etc. or may be floating point or real numbers like 2.4, 5.7, 14.8 etc. or may be the combination of both.
For example, if the user enters 10 and 15 as input, the program that adds two integer numbers will generate 25 as output since 10+15=25.
To view Flowchart click the link given below:
Add Two Integer Numbers Using a Third Variable
In this case two integer numbers given by the user are added and the result is stored in a third variable. Finally the result is displayed along with an appropriate message.
Program
#include
void main()
{
int num1,num2,add;
printf("Enter a number: ");
scanf("%d",&num1);
printf("Enter another number: ");
scanf("%d",&num2);
add=num1+num2;
printf("Sum is: %d",add);
}
Output
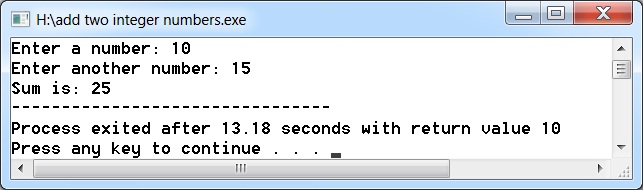
Explanation
- 3 integer variables namely num1, num2 and add are declared.
- The integer values entered by the user are stored in the variables num1 and num2Â respectively.
The values stored in num1 and num2 are added and the result is stored in the variable add.
- Finally the value of add is displayed along with an appropriate message.
Add Two Integer Numbers Without using a Third Variable
In this case two integer numbers given by the user are added and the result is directly displayed (without storing in a third variable) along with an appropriate message.
Program
#include
void main()
{
int num1,num2;
printf("Enter a number: ");
scanf("%d",&num1);
printf("Enter another number: ");
scanf("%d",&num2);
printf("Sum is: %d",num1+num2);
}
Output
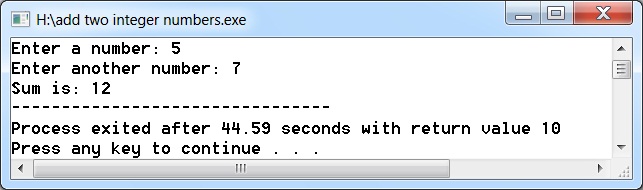
Explanation
- 2 integer variables namely num1 and num2Â are declared.
- The integer values entered by the user are stored in the variables num1 and num2Â respectively.
Finally the values stored in num1 and num2 are added and the result is directly displayed along with an appropriate message.
Add Two Floating Point or Real Numbers Using a Third Variable
Program
#include
void main()
{
float num1,num2,add;
printf("Enter a number: ");
scanf("%f",&num1);
printf("Enter another number: ");
scanf("%f",&num2);
add=num1+num2;
printf("Sum is: %f",add);
}
Output
Output 1
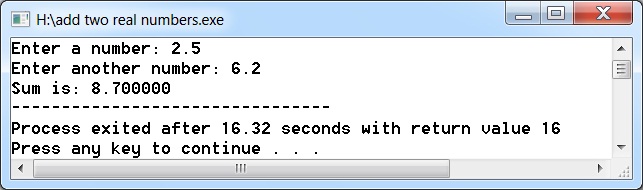
Output 2
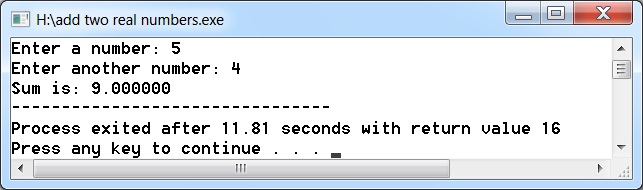
Output 3
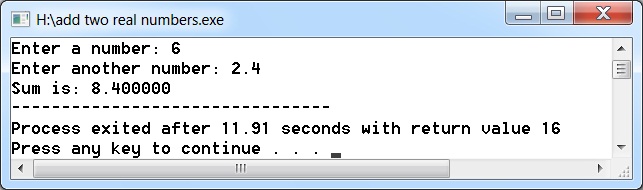
Explanation
- 3 float variables namely num1, num2 and add are declared.
- The numbers entered by the user are stored in the variables num1 and num2Â respectively.
The values stored in num1 and num2 are added and the result is stored in the variable add.
- Finally the value of add is displayed along with an appropriate message.
- This program is mainly written to add two floating point or real numbers.
- Though this program can also be used to add two integer numbers.
- Except that this program can also be used to add an integer number with a floating point or real number.
- If the user enters an integer number it will automatically be converted to a floating point or real number while storing the integer number to a float variable.
- This program can be modified to add two floating point or real numbers without using a third variable.
Add Two Integer Numbers using a Function
Program
#include
int sum(int n1,int n2);
void main()
{
int num1,num2,add;
printf("Enter a number: ");
scanf("%d",&num1);
printf("Enter another number: ");
scanf("%d",&num2);
add=sum(num1,num2);
printf("Sum is: %d",add);
}
int sum(int n1,int n2)
{
int a;
a=n1+n2;
return a;
}
Output
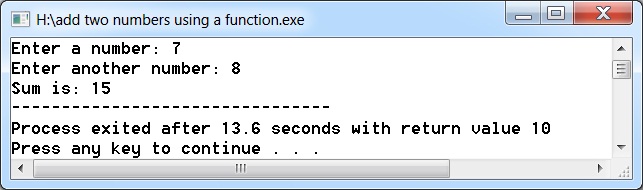
Explanation
- Inside main() method 3 integer variables namely num1, num2 and add are declared.
- The integer values entered by the user are stored in the variables num1 and num2 respectively inside main() method.
The values stored in num1 and num2 are passed to the function sum() which are stored in n1 and n2 respectively.
- Within sum() function an integer variable namely a is declared.
- The values stored in n1 and n2 are added and the sum is stored in a.
- Value of a is returned to the main() function and is stored in the variable add.
- Finally the value of add is displayed along with an appropriate message.
Add Two Integer Numbers using a Function (Alternate Program-1)
Program
#include
int sum(int n1,int n2);
void main()
{
int num1,num2,add;
printf("Enter a number: ");
scanf("%d",&num1);
printf("Enter another number: ");
scanf("%d",&num2);
add=sum(num1,num2);
printf("Sum is: %d",add);
}
int sum(int n1,int n2)
{
return n1+n2;
}
Output
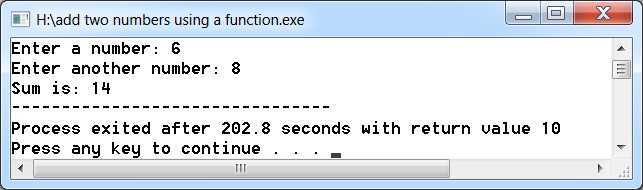
Explanation
- Inside main() method 3 integer variables namely num1, num2 and add are declared.
- The integer values entered by the user are stored in the variables num1 and num2 respectively inside main() method.
The values stored in num1 and num2 are passed to the function sum() which are stored in n1 and n2 respectively.
- Within sum() function the values stored in n1 and n2 are added and the sum is returned to the main() function and is stored in the variable add.
- Finally the value of add is displayed along with an appropriate message.
Add Two Integer Numbers using a Function (Alternate Program-2)
Program
#include
int sum(int n1,int n2);
void main()
{
int num1,num2;
printf("Enter a number: ");
scanf("%d",&num1);
printf("Enter another number: ");
scanf("%d",&num2);
printf("Sum is: %d",sum(num1,num2));
}
int sum(int n1,int n2)
{
return n1+n2;
}
Output
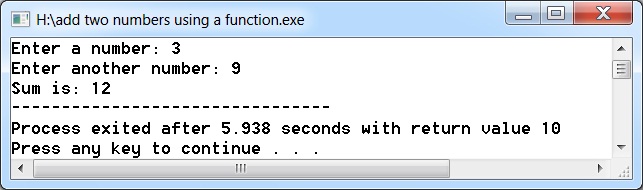
Explanation
- Inside main() method 2 integer variables namely num1 and num2Â are declared.
- The integer values entered by the user are stored in the variables num1 and num2 respectively inside main() method.
The values stored in num1 and num2 are passed to the function sum() which are stored in n1 and n2 respectively.
- Within sum() function the values stored in n1 and n2 are added and the sum is returned to the main() function and is directly displayed along with an appropriate message.