Menu
MENU
C - Count Number of Digits of a Number
Problem Statement
Write a program in C to count the number of digits present in an integer number entered by the user.
Brief Description
According to the problem statement we have to write a program in C that will take an integer value as input from the user through the keyboard and will count the total number of digits present in that number.
For example, if the user enters 132 as input, the program will generate 3 as output since the number 132 contains 3 digits.
Program
#include
void main()
{
int num,temp,count=0;
printf("Enter a number: ");
scanf("%d",&num);
temp=num;
do
{
count=count+1; //OR count++;
temp=temp/10; //OR temp/=10;
}while(temp!=0);
printf("Number of digits present in %d is %d",num,count);
}
Output
When the program given above is executed using Dev-C++ IDE we get the following output:
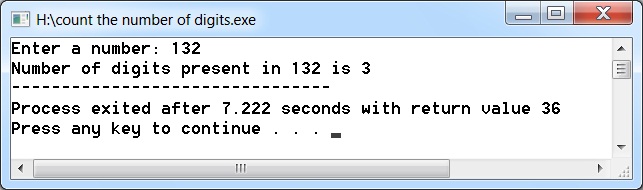
Explanation
In this program:
- 3 integer variable namely num, temp and count are declared.
- Initially 0 is stored in count.
- The integer value entered by the user is stored in num.
- A copy of the entered value is also stored in temp.
The step-by-step working of the do-while loop (when num and temp both contains 132) is shown in the table given below:
num | count=count+1; | count | temp=temp/10; | temp | temp!=0 | Comment |
---|---|---|---|---|---|---|
132 | ----- | 0 | ----- | 132 | Initial Values | |
132 | count=0+1; | 1 | temp=132/10; | 13 | 13!=0 ↓ 1(True) | Iteration 1 |
132 | count=1+1; | 2 | temp=13/10; | 1 | 1!=0 ↓ 1(True) | Iteration 2 |
132 | count=2+1; | 3 | temp=1/10; | 0 | 0!=0 ↓ 0(False) | Iteration 3 [do-while loop stops working as temp!=0 is evaluated to 0(False)] |
While executing the do-while loop:
- At first the value stored in count is incremented by 1
- After that the value stored in temp is divided by 10 to remove the last digit from the value stored in it and the result is stored in the same variable i.e. temp.
- At the end of each iteration value of temp is checked to see whether it has become 0 or not.
- The condition temp!=0 will produce either 1(True) or 0(False) after evaluation.
- If it is 1(True) the statements written within do-while loop are executed again and the loop continues to iterate till the condition is 1(True).
- If it is 0(False) then the loop stops working and the control goes to the statement written immediately after the loop.
Thus the above process is used to count the total number of digits present in the given number.
The final result is stored in count.Â
Finally the number of digits present in the entered integer value along with the value is displayed on the output screen.
Share this page on