Python - Add Two Numbers
Problem Statement
Brief Description
According to the problem statement we have to write a program in Python that will take two numbers as input from the user through the keyboard and will add the two given numbers and will find the sum.
The two numbers given by the user may be integers like 12, 15, 18 etc. or may be floating point or real numbers like 8.5, 12.7, 18.2 etc. or may be the combination of both.
For example, if the user enters 12 and 18 as input, the program that adds two integer numbers will generate 30 as output since 12+18=30.
To view Flowchart click the link given below:
Add Two Integer Numbers Using a Third Variable
In this case two integer numbers given by the user are added and the result is stored in a third variable. Finally the result is displayed along with an appropriate message.
Program
num1=int(input("Enter a number: "))
num2=int(input("Enter another number: "))
add=num1+num2
print("Sum is:",add)
Output
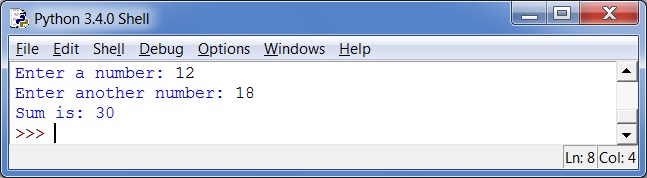
Explanation
- 3 variables namely num1, num2 and add are used.
- The integer values entered by the user are stored in the variables num1 and num2Â respectively.
The values stored in num1 and num2 are added and the result is stored in the variable add.
- Finally the value of add is displayed along with an appropriate message.
Add Two Integer Numbers Without using a Third Variable
In this case two integer numbers given by the user are added and the result is directly displayed (without storing in a third variable) along with an appropriate message.
Program
num1=int(input("Enter a number: "))
num2=int(input("Enter another number: "))
print("Sum is:",num1+num2)
Output
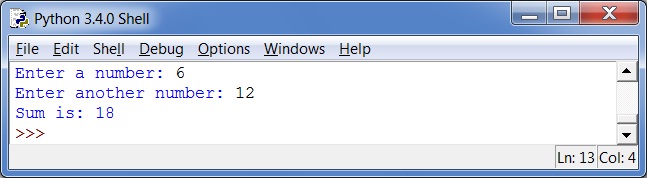
Explanation
- 2 variables namely num1 and num2 are used.
- The integer values entered by the user are stored in the variables num1 and num2Â respectively.
Finally the values stored in num1 and num2 are added and the result is directly displayed along with an appropriate message.
Add Two Floating Point or Real Numbers Using a Third Variable
Program
num1=float(input("Enter a number: "))
num2=float(input("Enter another number: "))
add=num1+num2
print("Sum is:",add)
Output
Output 1
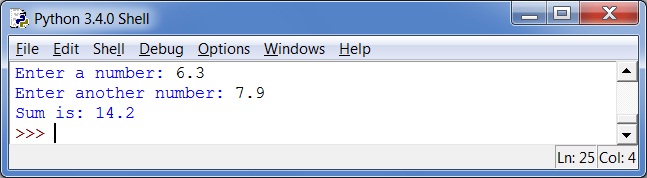
Output 2
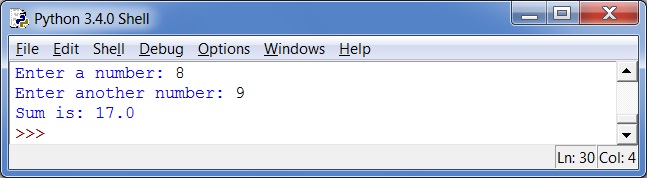
Output 3
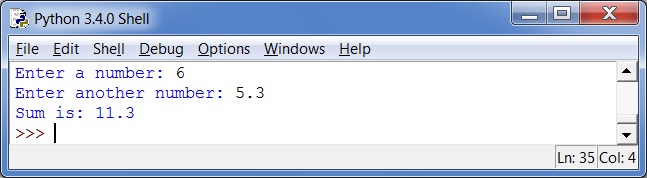
Explanation
- 3 variables namely num1, num2 and add are used.
- The numbers entered by the user are stored in the variables num1 and num2Â respectively.
The values stored in num1 and num2 are added and the result is stored in the variable add.
- Finally the value of add is displayed along with an appropriate message.
- This program is mainly written to add two floating point or real numbers.
- Though this program can also be used to add two integer numbers.
- Except that this program can also be used to add an integer number with a floating point or real number.
- If the user enters an integer number it will be converted to a floating point or real number by the function float().
- This program can be modified to add two floating point or real numbers without using a third variable.