C - Swapping of two numbers
Problem Statement
Brief Description
According to the problem statement we have to write a program in C that will take two numbers (in this program we will consider only integer numbers) as input from the user through the keyboard and will store in two different variables. After that we have to exchange the values stored in the two variables and finally have to print the result.Â
For example, if the user enters 5 and 10 as input and stores in 1st and 2nd variables respectively, the program will exchange the values stored in two variables and as a result value of 1st variable would become 10 and value of 2nd variable would become 5. Finally the values stored in both variables are displayed.
Swapping Using a Temporary or 3rd Variable
Program
#include
void main()
{
int num1,num2,temp;
printf("Enter a value to store in 1st variable: ");
scanf("%d",&num1);
printf("Enter a value to store in 2nd variable: ");
scanf("%d",&num2);
temp=num1;
num1=num2;
num2=temp;
printf("-----After Swapping-----");
printf("\nValue stored in 1st variable is: %d",num1);
printf("\nValue stored in 2nd variable is: %d",num2);
}
Output
Output 1
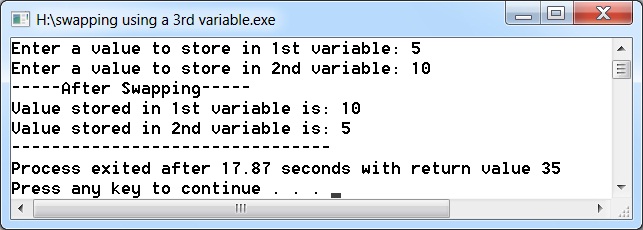
Output 2
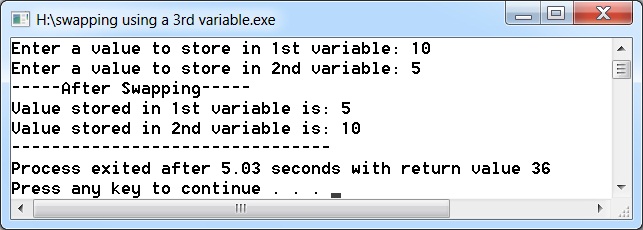
Explanation
- 3 integer variables namely num1, num2 and temp are declared.
- The integer values entered by the user are stored in the variables num1 and num2Â respectively.
A copy of the value stored in num1 is stored in temp.
After that a copy of the value stored in num2 is stored in num1.
Finally a copy of the value stored in temp is stored in num2.
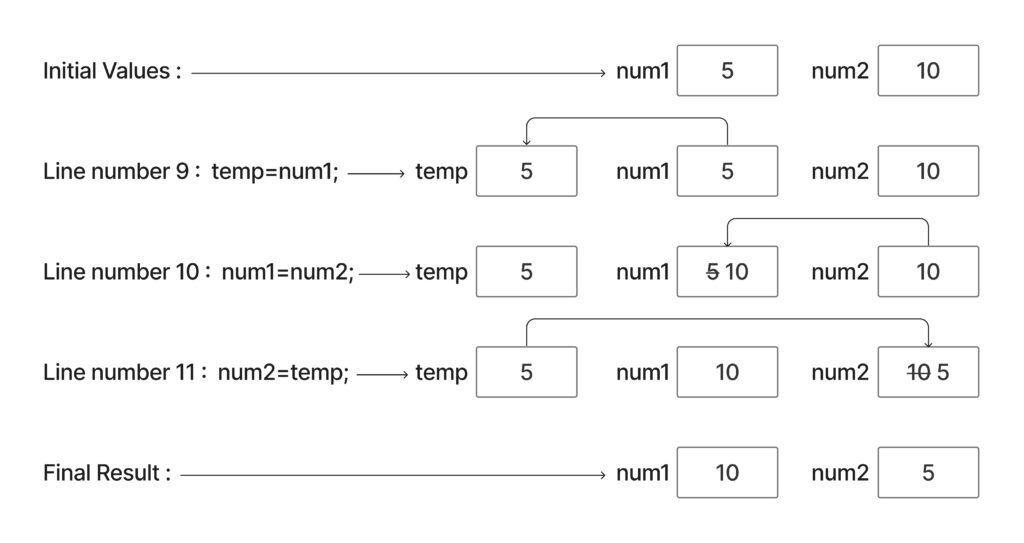
Swapping Without Using a Temporary or 3rd Variable
Program
#include
void main()
{
int num1,num2;
printf("Enter a value to store in 1st variable: ");
scanf("%d",&num1);
printf("Enter a value to store in 2nd variable: ");
scanf("%d",&num2);
num1=num1+num2;
num2=num1-num2;
num1=num1-num2;
printf("-----After Swapping-----");
printf("\nValue stored in 1st variable is: %d",num1);
printf("\nValue stored in 2nd variable is: %d",num2);
}
Output
Output 1
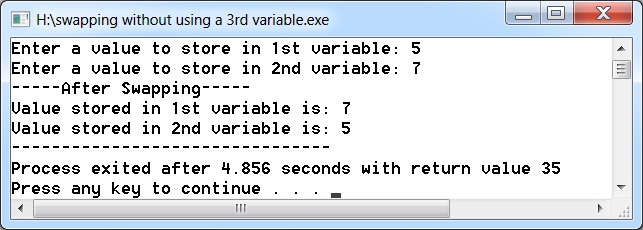
Output 2
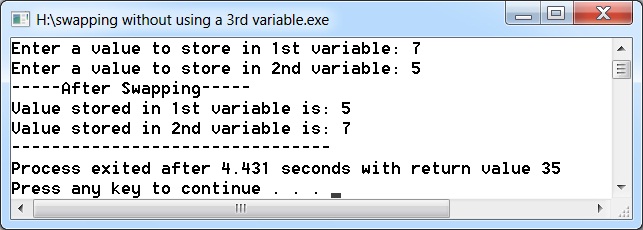
Explanation
- 2 integer variables namely num1 and num2Â are declared.
- The integer values entered by the user are stored in the variables num1 and num2Â respectively.
- The values stored in num1 and num2 are added and the sum is stored in num1.
- The value stored in num2 is subtracted from the value stored in num1 (which contains the sum of num1 and num2) and the result is stored in num2.
- Finally the value stored in num2 is subtracted from the value stored in num1 (which contains the sum of num1 and num2) and the result is stored in num1.
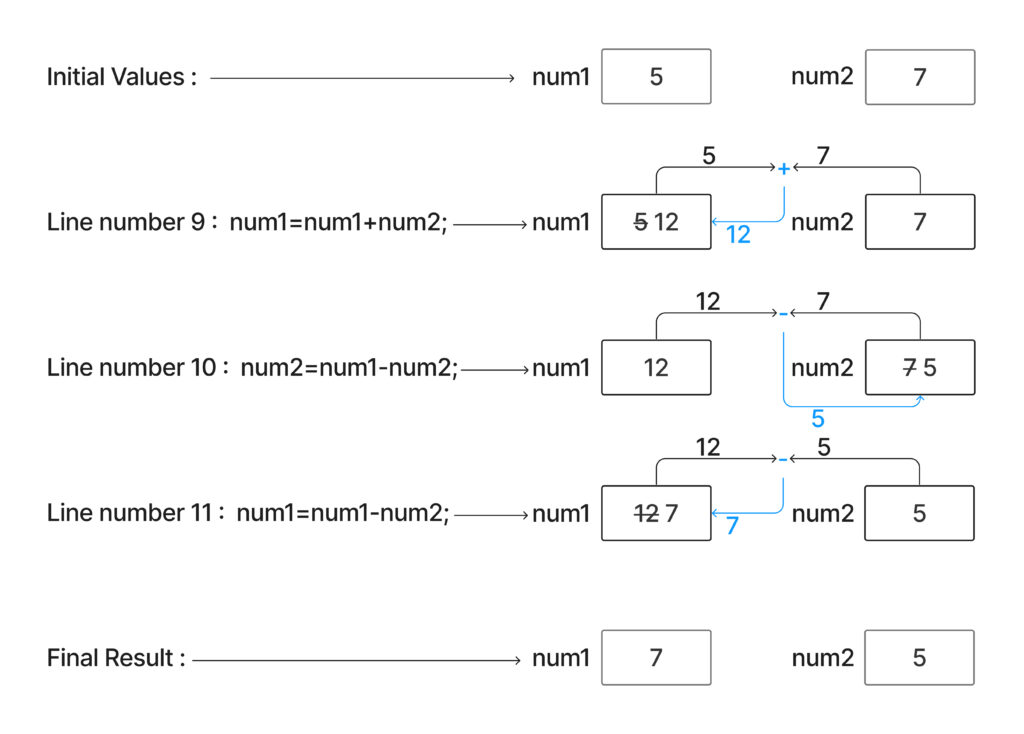